CS 144 Checkpoint 2 - TCP Receiver
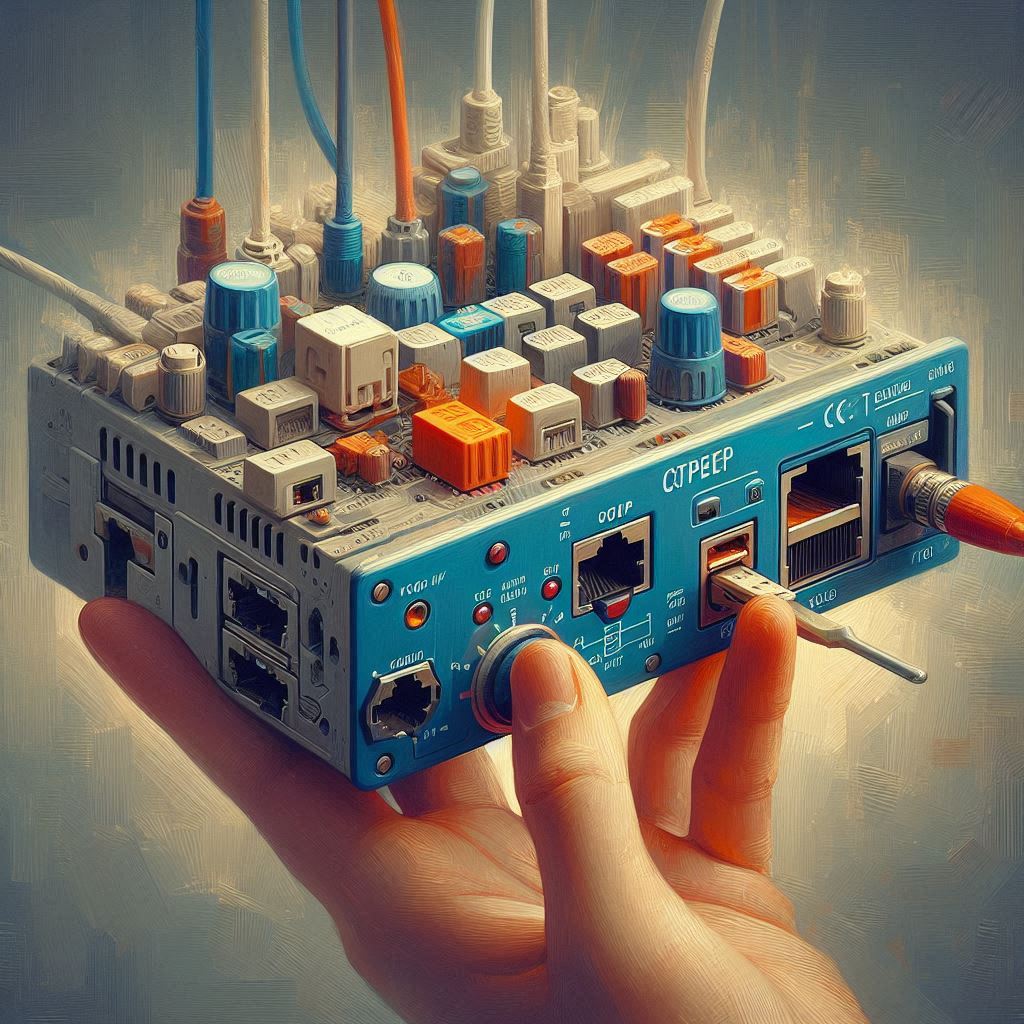
“In this lab you’ll implement the “receiver” part of TCP, responsible for receiving messages from the sender, reassembling the byte stream (including its ending, when that occurs), and determining messages that should be sent back to the sender for acknowledgment and flow control.”
Sequence number translation
The Sequence Number is a sequence that satisfies
- where is a random 32-bit unsigned integer.
- for .
The Absolute Sequence Numbers is a sequence that satisfies for all .
Given an Absolute Sequence Number , the corresponding Sequence Number
Conveniently, unsigned integer addition overflow is well defined in C++ and it behaves exactly like the modulo operation. Therefore, the implementation of Wrap32::wrap
is simple:
Wrap32 Wrap32::wrap( uint64_t n, Wrap32 zero_point )
{
return zero_point + n;
}
The inverse of the operation is more difficult. Given a Sequence Number , we cannot uniquely determine the index , because
To uniquely determine the index, we are also given a checkpoint . We need to find such that and is minimised. We have
To find , we have the following algorithm. We first find the smallest inside the set , if it is greater than , then we are done, because anything greater than will have a larger distance to . If is less than , we find and such that , as two candidates for . We then compare and and choose the smaller one as .
TCP receiver
There isn’t much to talk about inside the TCP receiver. Checkout the test cases if anything is unclear in the specification.
This concludes Checkpoint 2.